idea新建多模块工程
idea新建多模块工程
创建继承关系模块(两个模块具有父子关系)
创建父子模块
第一步:创建父模块
依次点击 File -> New -> Project ->Maven ->Next


创建后目录结构如下:
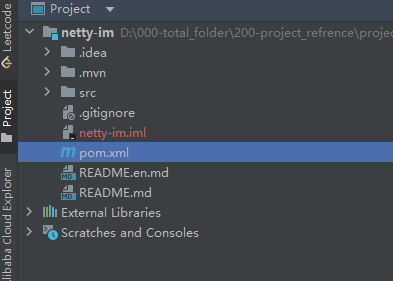
第二步:修改父模块pom
在修改pom文件之前,先删掉父模块中的src目录。
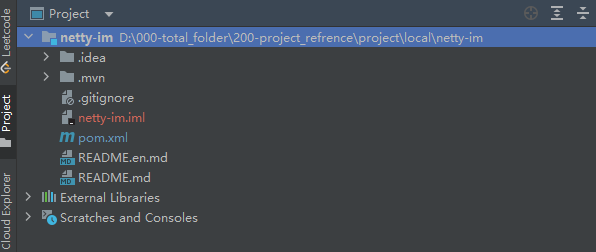
然后在修改pom文件,如果只是简单能用,其实只要修改如下两个配置即可:
1、添加标签并将其改为pom。
2、添加标签配置对所有模块依赖的管理。
修改后完整pom如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63
| <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId> <artifactId>netty-im</artifactId> <version>0.0.1-SNAPSHOT</version>
<packaging>pom</packaging>
<modules> <module>redis-learn</module> <module>pro-common</module> </modules>
<properties> <maven.compiler.source>8</maven.compiler.source> <maven.compiler.target>8</maven.compiler.target> </properties>
<dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> <version>2.3.3.RELEASE</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <version>2.3.3.RELEASE</version> <scope>compile</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-redis</artifactId> <version>2.3.3.RELEASE</version> </dependency> </dependencies> </dependencyManagement> <dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-dependencies</artifactId> <version>${spring-boot.version}</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement>
</project>
|
创建子模块
第一步:创建子模块
选中父工程 pro-learn -> New -> Module -> Spring Initializr -> Module SDK(选择自己的jdk版本) -> Next

按自己需求填写如下信息,点击Next进入下一步。
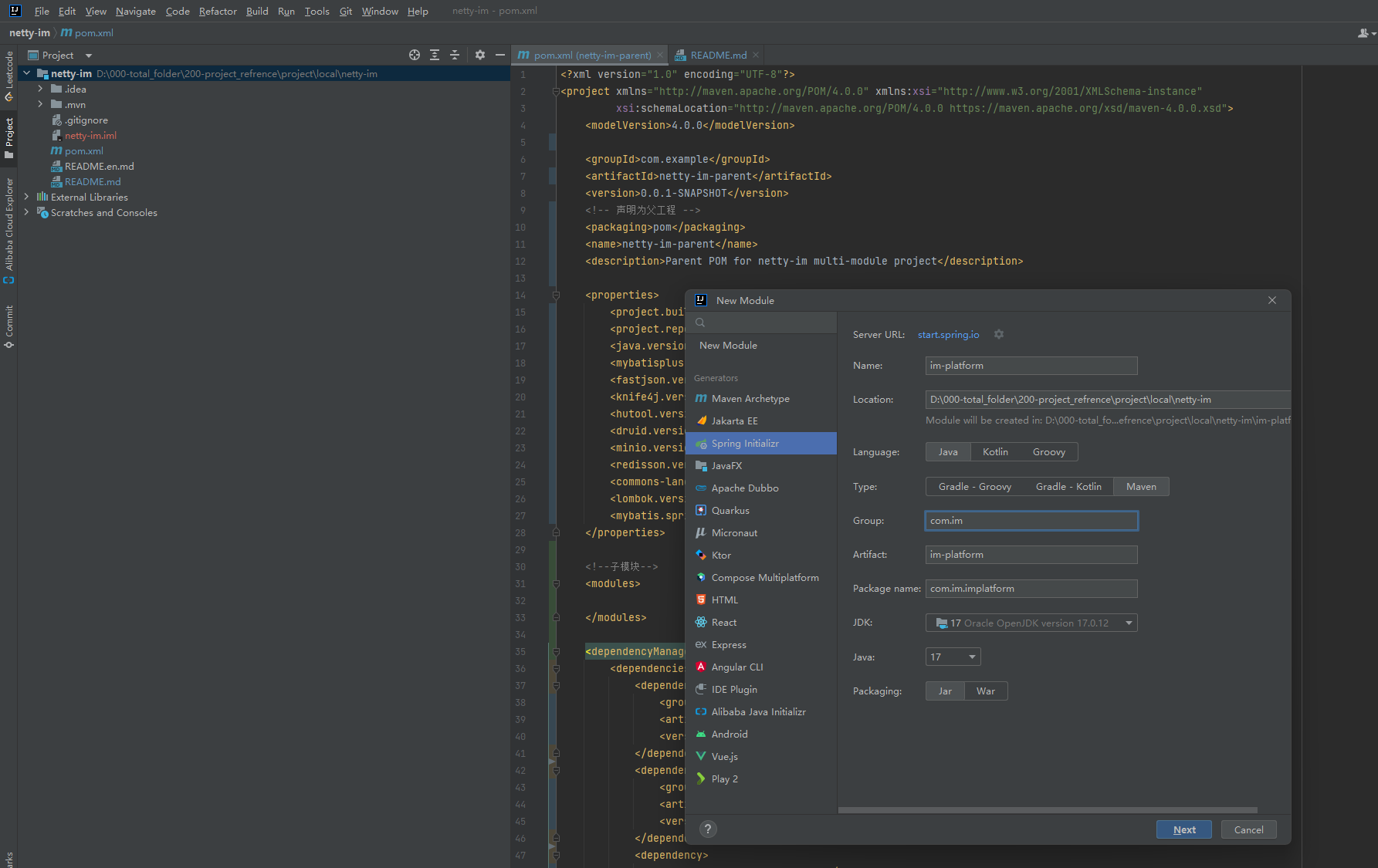
这里可以什么都不选,点击Next进入下一步,然后,按自己需求填写信息,最后点击create完成子模块的创建。
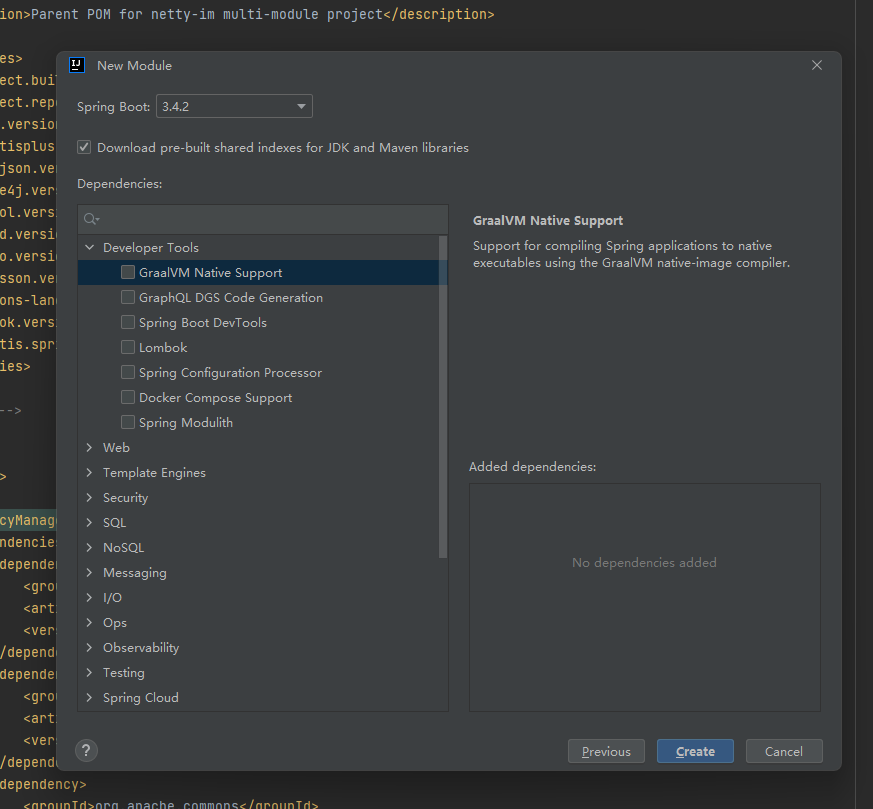
创建子模块后的目录结构如下:
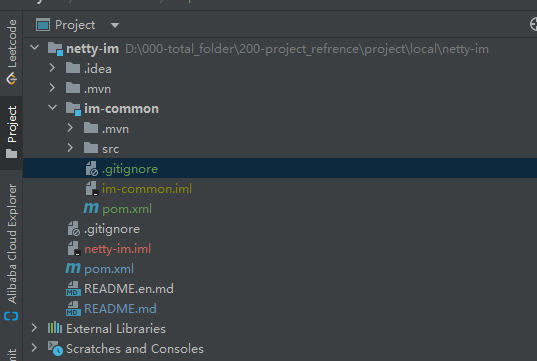
第二步:父模块中添加modules管理子模块
在父pom中添加如下配置:
1 2 3 4
| <modules> <module>im-common</module> </modules>
|
第三步:配置子模块pom
将redis-learn模块pom文件中parent中的坐标(groupId、artifactId、version)改为父模块的坐标,及添加依赖,具体看以下pom文件中的注释:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>com.im</groupId> <artifactId>netty-im-parent</artifactId> <version>0.0.1-SNAPSHOT</version> </parent>
<modelVersion>4.0.0</modelVersion> <artifactId>im-common</artifactId> <packaging>jar</packaging>
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> </dependency> </dependencies>
<build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build>
</project>
|
创建依赖关系模块(一个模块引用另一个模块)
在我们开发中,是不是经常把一些公用的方法,比如你的util工具类,常量类等放到一个单独的包中,比如放到common包中,如果分多模块,就可以把这些公共方法单独放到一个模块中。
创建子模块
第一步:创建子模块
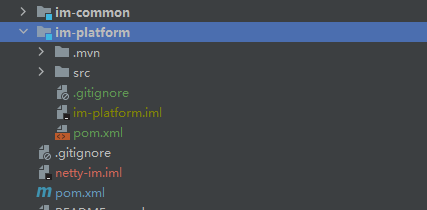
修改im-platform模块中的pom文件,通过pro-common的坐标来引用im-common模块,在im-platform的pom中添加如下配置:
1 2 3 4 5 6
| <dependency> <groupId>com.im</groupId> <artifactId>im-common</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency>
|
至此,一个基本的maven管理的多模块项目就搭建完毕了。